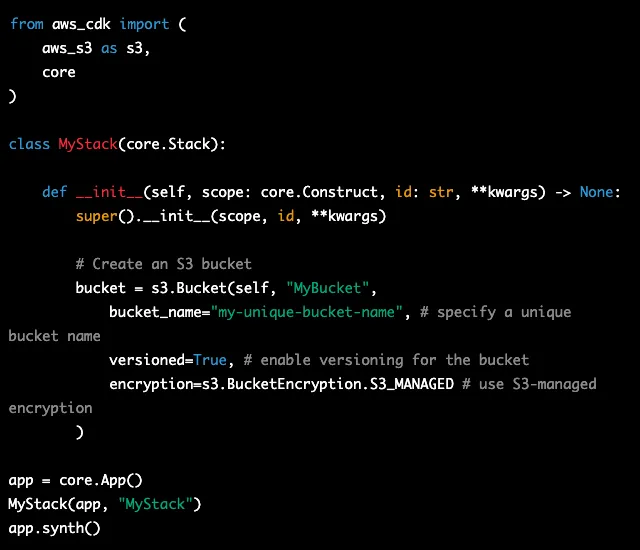
How to create an Amazon S3 bucket using AWS CDK (Cloud Development Kit) in Python ?
from aws_cdk import (
aws_s3 as s3,
core
)
class MyStack(core.Stack):
def __init__(self, scope: core.Construct, id: str, **kwargs) -> None:
super().__init__(scope, id, **kwargs)
# Create an S3 bucket
bucket = s3.Bucket(self, "MyBucket",
bucket_name="my-unique-bucket-name", # specify a unique bucket name
versioned=True, # enable versioning for the bucket
encryption=s3.BucketEncryption.S3_MANAGED # use S3-managed encryption
)
app = core.App()
MyStack(app, "MyStack")
app.synth()
This code creates a new stack called MyStack and in the constructor of the stack we are creating a new S3 bucket using the s3.Bucket class. We are passing the following properties to the bucket:
bucket_name
: a unique name for the bucket, this name should be unique among all S3 bucket names in the entire AWS account.versioned
: enable versioning for the bucket, this allows multiple versions of an object to be stored in the bucket.encryption
: use S3-managed encryption for the bucket, this encrypts the objects stored in the bucket using server-side encryption.
Finally, the last line of the script app.synth()
is used to create the stack and create the S3 bucket in your AWS account.
It’s important to note that this code only creates the S3 bucket and does not define any permissions or access controls for the bucket. In a real-world scenario, you would likely want to add additional resources and configure access controls to the bucket to meet your security requirements.
More Articles
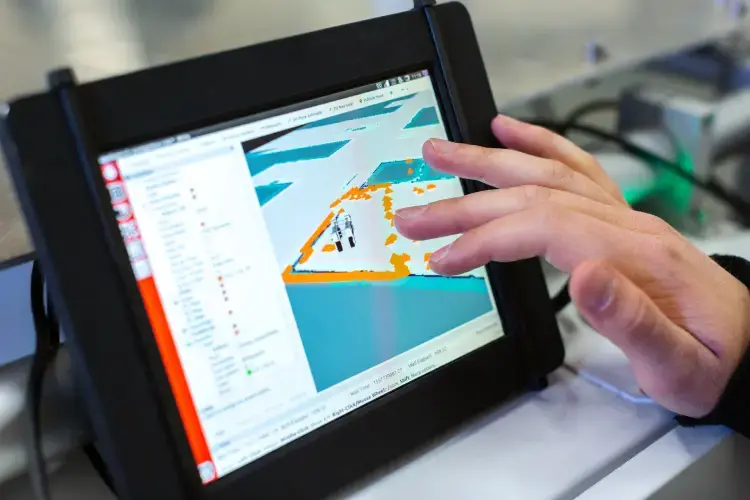
Benefits of QA Testing - Manual and Automation
Quality Assurance (QA) testing is a critical component of the software development process. It ensures that software products meet the highest standards of quality, reliability, and user satisfaction. QA testing can be approached through manual testing, where human testers perform various test cases, or automation testing, which utilizes software tools and scripts to execute tests automatically. In this article, we'll explore the benefits of both manual and automation QA testing.
Read all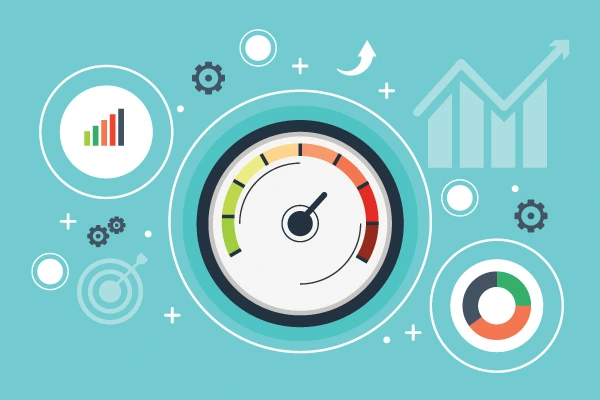
Demystifying Performance Testing: Best Approach, Tools, and Benefits
In today's fast-paced digital landscape, performance is a critical factor that directly impacts user satisfaction and business success. Performance testing plays a crucial role in ensuring that software applications meet performance expectations under various conditions. In this article, we'll explore the best approach to performance testing, popular tools, and the benefits it brings to the table.
Read all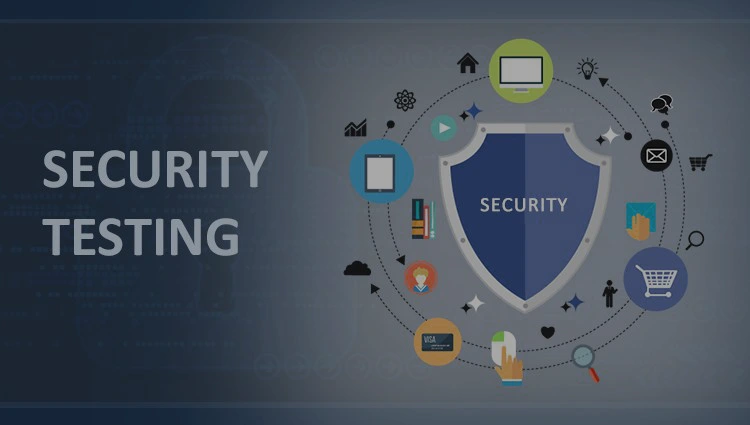
QA Perspective on Security Testing
In today's interconnected world, software security is of paramount importance. The rising number of cyber threats and data breaches underscores the critical need for robust security measures. As part of the Quality Assurance (QA) process, security testing plays a crucial role in identifying vulnerabilities and ensuring the resilience of software applications. In this article, we'll explore security testing from a QA perspective and highlight its significance in safeguarding software systems.
Read all